Objectifs
On souhaite réaliser des mesures de température / humidité des sols et exposer ces données via une API web. Pour cela on va utiliser une sonde SHT10 et une Arduino uno+Ethernet shield.
Liste matériel :
- - 1 Arduino Uno + Alim. + Cable USB
- - 1 Arduino Ethernet Shield
- - 1 sonde SHT10
- - 1 cable RJ45
- - 1 breadboard
- - 1 résistance de 10 kOhm
- - fils breadboard
- - cable électrique multi-brins Ø 0.25mm [couleurs différentes]
- - connecteurs clip ou fer à souder
Sonde SHT10 :
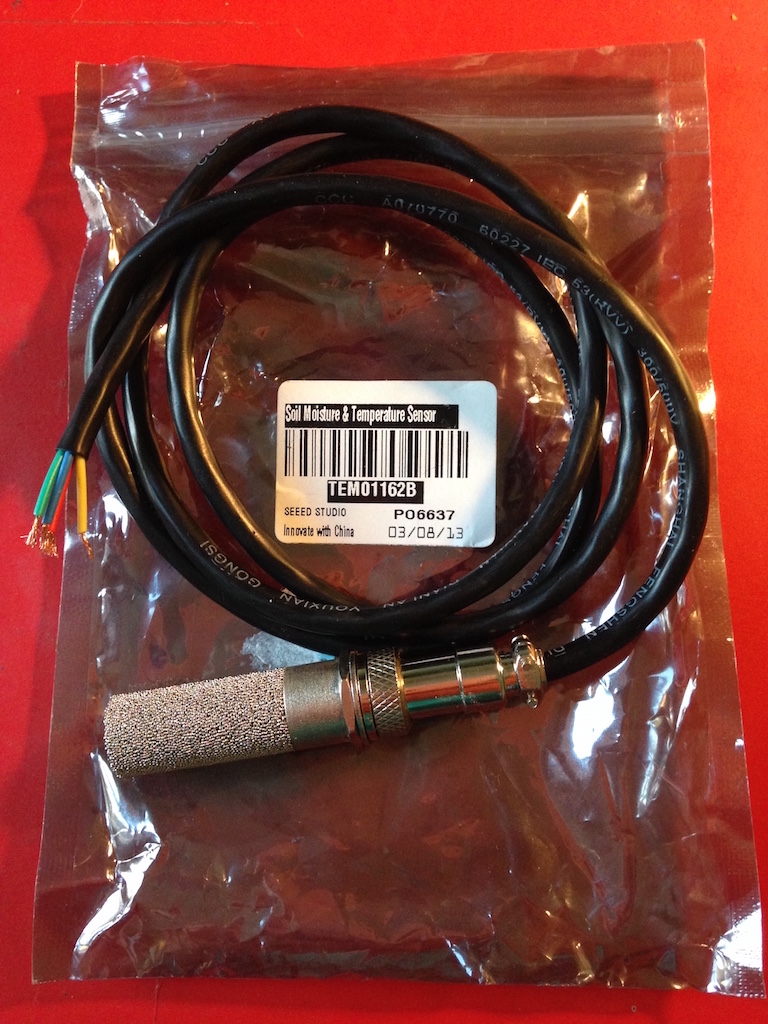
1 - Import de la librairie
Récupérer la librairie sur github :
https://github.com/practicalarduino/SHT1xCopier le contenu du dossier dans ~/Documents/Arduino/librairies/SHT11/
Créer le dossier SHT11 auparavant.
2 - Connexion de la sonde à la bread board
Le connexion de la sonde dispose de la nomenclature suivante :
- - rouge : vcc +3,3v ou +5v
- - vert : gnd
- - bleu : data - digital 8
- - jaune : horloge - digital 9
Connexion Arduino :
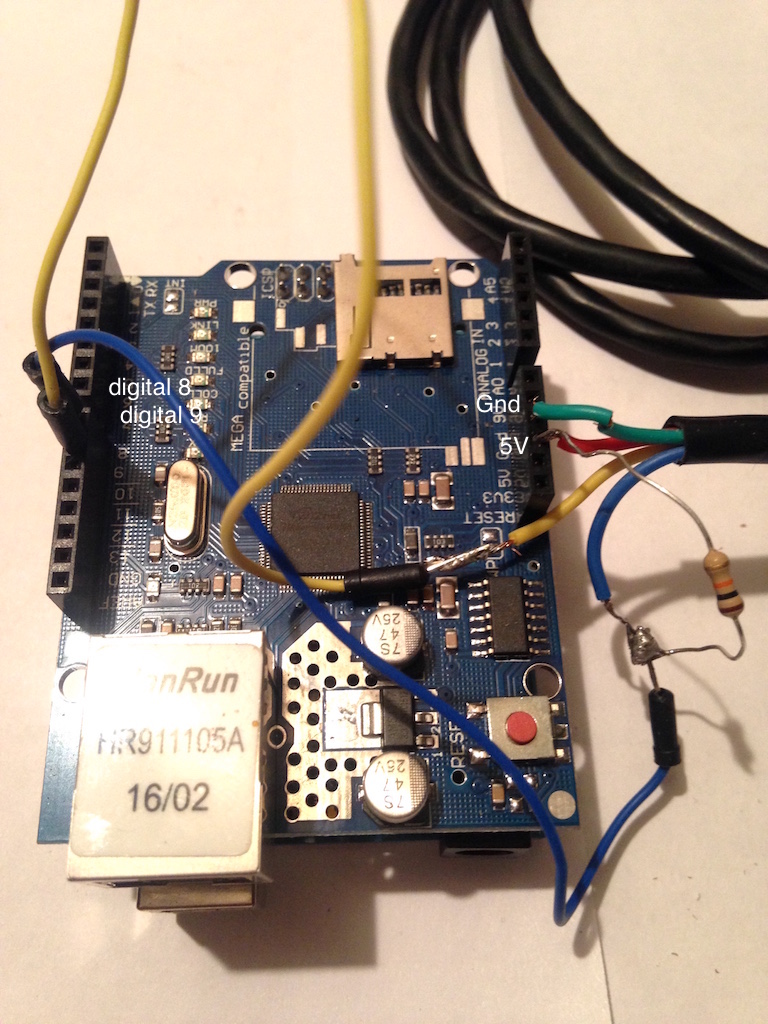
Il est nécessaire de connecter une résistance de 10 kOhm entre le pôle positif (rouge) et le canal data (bleu).
Connecter l'Arduino au port usb de notre ordinateur.
3 - Script de capture des valeurs
On lance l'IDE Arduino et on choisit dans le menu "Ouvrir" dans la barre d'outils : /librairies/SHT11/ReadSHT1xValues
Télécharger le programme dans l'Arduino et test le script avec le moniteur série en choisissant la vitesse : 38400 baud.
#include <SHT1x.h>
#include <Ethernet.h>
#include <SPI.h>
// Specify data and clock connections and instantiate SHT1x object
#define dataPin 10
#define clockPin 11
SHT1x sht1x(dataPin, clockPin);
void setup()
{
Serial.begin(38400); // Open serial connection to report values to host
Serial.println("Starting up");
}
void loop()
{
float temp_c;
float temp_f;
float humidity;
// Read values from the sensor
temp_c = sht1x.readTemperatureC();
temp_f = sht1x.readTemperatureF();
humidity = sht1x.readHumidity();
// Print the values to the serial port
Serial.print("Temperature: ");
Serial.print(temp_c, DEC);
Serial.print("C / ");
Serial.print(temp_f, DEC);
Serial.print("F. Humidity: ");
Serial.print(humidity);
Serial.println("%");
delay(2000);
}
Version du script renvoyant un objet json en http depuis l'ethernet shield :
Attention à ne pas utiliser les digital pins 10 et 11 qui sont utilisé par l'ethernet shield.
#include <SHT1x.h>
#include <Ethernet.h>
// Specify data and clock connections and instantiate SHT1x object
#define dataPin 8
#define clockPin 9
SHT1x sht1x(dataPin, clockPin);
// Enter a MAC address and IP address for your controller below.
// The IP address will be dependent on your local network:
byte mac[] = {
0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEF
};
IPAddress ip(192, 168, 0, 12);
// Initialize the Ethernet server library
// with the IP address and port you want to use
// (port 80 is default for HTTP):
EthernetServer server(80);
void setup(){ // Initialisation
Serial.begin(9600); //Connection série à 38400 baud
// start the Ethernet connection and the server:
Ethernet.begin(mac, ip);
server.begin();
Serial.print("server is at ");
Serial.println(Ethernet.localIP());
}
void loop() { //boucle principale
// listen for incoming clients
EthernetClient client = server.available();
if (client) {
Serial.println("new client");
// an http request ends with a blank line
boolean currentLineIsBlank = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
// if you've gotten to the end of the line (received a newline
// character) and the line is blank, the http request has ended,
// so you can send a reply
if (c == '\n' && currentLineIsBlank) {
// read values from sensor
int temp_c;
int temp_f;
float humidity;
// Read values from the sensor
temp_c = sht1x.readTemperatureC();
temp_f = sht1x.readTemperatureF();
humidity = sht1x.readHumidity();
Serial.println(sht1x.readTemperatureC());
Serial.println(sht1x.readHumidity());
// send a standard http response header
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: application/json");
client.println("Connection: close"); // the connection will be closed after completion of the response
//client.println("Refresh: 5"); // refresh the page automatically every 5 sec
client.println();
client.print("{\"data\":");
client.print("{\"temperature\": ");
client.print(temp_c, DEC);
client.print(",");
client.print("\"hydrometry\": ");
client.print(humidity);
client.print("}");
client.print("}");
break;
}
}
}
// give the web browser time to receive the data
delay(1);
// close the connection:
client.stop();
Serial.println("client disconnected");
}
}